SDK integration for password update
DataDome Account Protect detects account takeover threats and protects you against them
Account Protect can be integrated into your backend through SDK packages available on multiple platforms.
Prerequisites for Account Protect
Account Protect is separate from Bot Protect and is not available on your account by default.
Please contact your account manager to enable it.This service requires a dedicated API key, which will be available on your dashboard once it is enabled.
Main concepts
DataDome Account Protect safeguards all password updates—whether user-initiated, recovery-based, or merchant-forced—by analyzing each attempt and recommending whether to allow or deny it. This real-time protection ensures that only legitimate users can change account credentials, reducing the risk of account takeover and credential abuse.
DataDome supports three customer journeys:
- When a user has forgotten their password (User Journey 1)
- When a user wants to update their password (User Journey 2)
- When the merchant forces a user to reset their password (User Journey 3)
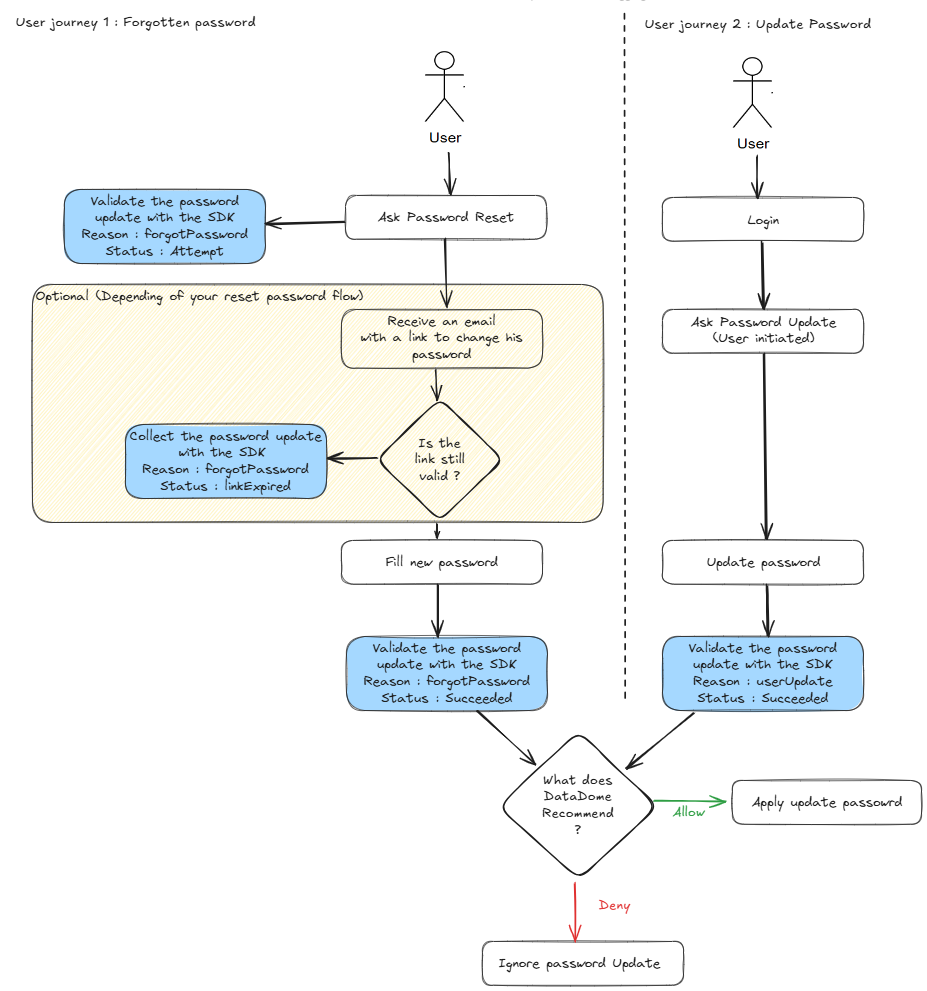
Overview of the implementation flow for a Password update. (User Journey 1 & 2)

Overview of the implementation flow for Password update (User journey 3)
Installation
The Account Protect SDK is distributed on multiple platforms:
- Maven package for Java 11+ and Spring Boot 2.x
- Maven package for Java 17+ and Spring Boot 3.x - compatible with Scala 2.12+
- Npm package for Node.js 14 or newer
- NuGet package for .NET 6
- Packagist package for Symfony PHP (8.1+) applications
- Packagist package for Laravel PHP (8.1+) applications
- Pypi package for Python 3.8 +
- Ruby gem for Ruby 3.2+
- Go package for Go 1.18+
You can use one of the commands below to install the relevant package for your application:
npm i @datadome/fraud-sdk-node
dotnet add package DataDome.AspNetCore.Fraud.SDK
<!-- insert in the pom.xml file of the project -->
<dependency>
<groupId>co.datadome.fraud</groupId>
<artifactId>fraud-sdk-java</artifactId>
<!-- <version>1.0.1</version> --> <!-- compatible with Spring Boot 2.x -->
<version>2.2.2</version> <!-- compatible with Spring Boot 3.x -->
</dependency>
libraryDependencies += "co.datadome.fraud" % "fraud-sdk-java" % "2.2.1"
pip install datadome-fraud-sdk-python
composer require datadome/fraud-sdk-symfony
# 1. add `datadome/fraud-sdk-laravel` to your project
composer require datadome/fraud-sdk-laravel
# 2. Generate an autoloader
composer dump-autoload
# 3. Edit `config/app.php` to add `DataDomeServiceProvider`
# config/app.php
use DataDome\FraudSdkLaravel\Providers\DataDomeServiceProvider;
[...]
'providers' => ServiceProvider::defaultProviders()->merge([
[...]
DataDomeServiceProvider::class
# 4. publish `datadome.php` in the `config` folder
php artisan vendor:publish
gem install datadome_fraud_sdk_ruby
go get github.com/datadome/fraud-sdk-go-package
Usage
Using the Account Protect SDK requires changes in your application to send signals regarding password updates and handle the recommendations provided by DataDome's Account Protect API.
Example for an Password Update event
package main
import (
"log"
"net/http"
"time"
dd "github.com/datadome/fraud-sdk-go"
)
func passwordHandler(client *dd.Client) http.HandlerFunc {
return func(w http.ResponseWriter, r *http.Request) {
if r.Method == http.MethodPut {
email := "[email protected]"
user := dd.User{
ID: "fake_user_id",
}
createdAt := time.Now().Format(time.RFC3339)
sessionId := "fake_session_id"
session := dd.Session{
ID: &sessionId,
CreatedAt: &createdAt,
}
reason := dd.UserUpdate
status := dd.PasswordUpdateSucceeded
validate, err := client.Validate(r, dd.NewPasswordUpdateEvent(
email,
user,
reason,
status,
dd.PasswordUpdateWithSession(session),
))
if err != nil {
log.Printf("error during validation: %v\n", err)
}
if validate.Action == dd.Allow {
w.WriteHeader(http.StatusOK)
return
} else {
// Business Logic here
// MFA
// Challenge
// Notification email
// temporarly lock account
http.Error(w, "failed", http.StatusForbidden)
return
}
}
}
}
func main() {
client, _ := dd.NewClient("FRAUD_API_KEY")
mux := http.NewServeMux()
mux.HandleFunc("/password", passwordHandler(client))
_ = http.ListenAndServe(":8080", mux)
}
API Reference
PasswordUpdateEvent
PasswordUpdateEvent
The SDK exposes methods to validate password updates that require a PasswordUpdateEvent
instance to be sent to the Account Protect API along with the client request itself.
Available properties for this event type are listed below:
Name | Description | Default value | Possible values | Optional |
---|---|---|---|---|
account | The unique account identifier used for the login attempt. | Any string value. | No | |
reason | Reason for the password change. | forcedReset, forgotPassword, userUpdate | No | |
session.createdAt | Creation date of the session | Format ISO 8601 YYYY-MM-DDThh:mm:ssTZD | Yes | |
session.id | A unique session identifier from your system | Any string value. | Yes | |
status | Status of the password change | attempt, failed, succeeded, linkExpired | ||
user.id | A unique customer identifier from your system. It has to be the same for all other events sent | Any string value. | No |
Validation response
Validating a password update event should result in a response that can include the following properties:
Name | Description | Possible Values | Always Defined |
---|---|---|---|
action | The recommended action to perform on the login attempt. | allow , deny | Yes |
errors | A list of objects representing each error with details. Each object will have the properties listed below. | ||
errors[i].error | A short description of the error. | ||
errors[i].field | The name of the value that triggered the error. | ||
eventId | Event identifier associated to this validate event. | A valid UUID. | Yes |
message | A description of the error if the status is failure or timeout . | Invalid header / Request timed out... | |
reasons | A list of reasons to support the recommended action. | List of reasons (Any string value.) | |
score | The level of confidence when identifying a request as coming from a fraudster. Only available in Ruby SDK 2.1.0+ and Go SDK v1.1.0+ | Integer | |
status | The status of the request to the fraud protection API. | ok , failure , timeout | Yes |
Options
Options can be applied to the SDK during its instantiation.
Option Name | Description | Default Value |
---|---|---|
endpoint | The endpoint to call for the Account Protect API. | https://account-api.datadome.co |
timeout | A timeout threshold in milliseconds. When an API request times out, the SDK will allow it by default. | 1500 |
You can find usage examples for each platform below:
client, err := dd.NewClient(
"FRAUD_API_KEY",
dd.ClientWithEndpoint("account-api.datadome.co"),
dd.ClientWithTimeout(1500),
)
FAQ
What happens if there is a timeout on API request?
The SDK has been designed to have minimal impact on the user experience. If the configured timeout is reached, the SDK will cancel its pending operation and allow the application to proceed.
What happens if the API returns an error?
Errors and timeouts are handled the same way by the SDK: it will not interrupt the application and allow it to proceed.
What happens if my API key is incorrect?
Invalid keys are detected when calling the account protect API. The SDK will return an allow
response to avoid blocking any login or registration attempt on the application. This response will also have a failure
status and a message that describes the problem.
Updated about 1 month ago