Auth0 integration
DataDome Account Protect detects account takeover threats and fake registration and protects you against them
Prerequisites for Account Protect
Account Protect is separate from Bot Protect and is not available on your account by default.
Please contact your account manager to enable it.This service requires a dedicated API key, which will be available on your dashboard once it is enabled.
Main concepts
Setting up Account Protect on Auth0 will enable us to collect more business data (email, social ID, ...) during login and registration events. We will be able to detect account takeovers and fake account creations.
You can find more insights about our detection on the Account Protect Dashboard.
Installation
Step 1: Configure Auth0 to send successful login events to DataDome Account Protect
- Connect to the Auth0 Console
- Go to Actions > Library and click on Create Action > Build from scratch
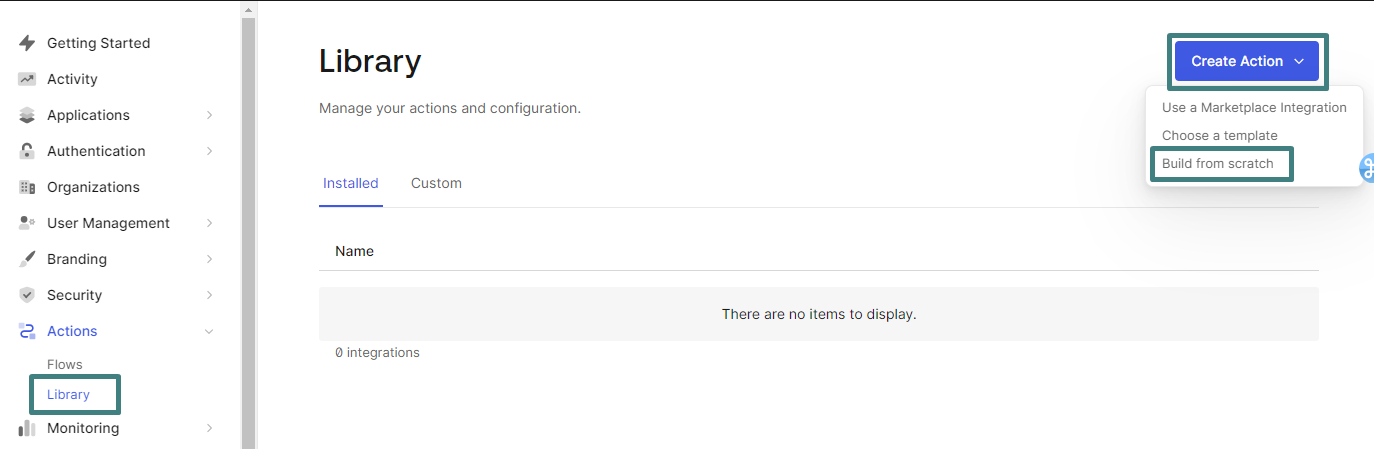
- Enter Account Protect - Login for the name, select Login / Post Login for the trigger and Node 18 for the runtime and click on Create
- Copy / Paste the following code and replace
FRAUD_API_KEY
on line 37 with your Account Protect Key available in your Dashboard
const axios = require('axios');
/**
* Handler that will be called during the execution of a PostLogin flow.
*
* @param {Event} event - Details about the user and the context in which they are logging in.
* @param {PostLoginAPI} api - Interface whose methods can be used to change the behavior of the login.
*/
exports.onExecutePostLogin = async (event, api) => {
// Filter refresh token event to not send these events to Athos
if (event.request.query?.prompt === 'none' || event.transaction?.protocol === 'oauth2-refresh-token') {
return;
}
const payload = {
event: "login",
account: event.user.email ?? event.user.user_id,
status: "succeeded",
module: {
requestTimeMicros: new Date().getTime()*1000,
name: "Fraud SDK Auth0",
version: "1"
},
header: {
addr: event.request.ip,
method: event.request.method,
host: event.request.hostname,
port: 443,
protocol: "https",
userAgent: event.request.user_agent,
clientID: event.client.client_id
}
};
const config = {
headers: {
'x-api-key': 'FRAUD_API_KEY',
'Content-type': 'application/json'
},
timeout: 1500,
};
try {
const res = await axios.post('https://account-api.datadome.co/v1/validate/login', payload, config);
if (res.status === 200 && res.data?.action === 'deny') { // deny login only if return 200 & action = deny.
// Custom actions
}
} catch (error) {
console.log(error);
}
};
We need to add a dependency to Axios, as we use it as the HTTP client.
- Click on Add Dependency
- Enter
axios
in the name fielc and click on Create
- Configuration is now done and you can deploy this new action to your tenant
- Click on Deploy
Now you have to use the new action (Account Protect - Login) in your authentication pipeline
- Go to Actions > Flows
- Click on Login
- Click on Custom on the right panel and move your action in your pipeline
- Click on Apply
From now on, you will send all succesful logins to DataDome Account Protect.
Step 2: Configure Auth0 to send registration events to DataDome Account Protect
- Connect to the Auth0 Console
- Go to Actions > Library and click on Create Action > Build from scratch
-
Enter Account Protect - Registration for the name, select Pro User Registration for the trigger and Node 18 for the runtime and click on Create
-
-
Copy / Paste the following code and replace
FRAUD_API_KEY
on line 37 with your Account Protect Key available in your Dashboard
/**
* Handler that will be called during the execution of a PreUserRegistration flow.
*
* @param {Event} event - Details about the context and user that is attempting to register.
* @param {PreUserRegistrationAPI} api - Interface whose methods can be used to change the behavior of the signup.
*/
const axios = require("axios");
exports.onExecutePreUserRegistration = async (event, api) => {
const payload = {
account: event.user.email ?? event.user.user_id,
status: "succeeded",
module: {
requestTimeMicros: new Date().getTime()*1000,
name: "Fraud SDK Auth0",
version: "1"
},
header: {
addr: event.request.ip,
method: event.request.method,
host: event.request.hostname,
port: 443,
protocol: "https",
userAgent: event.request.user_agent,
clientID: event.client.client_id
},
user: {
id: event.user.email ?? event.user.user_id,
email:event.user.email
}
};
const config = {
headers: {
'x-api-key': 'FRAUD_API_KEY',
'Content-type': 'application/json'
},
timeout: 1500,
};
try {
const res = await axios.post('https://account-api.datadome.co/v1/validate/registration', payload, config);
if (res.status === 200 && res.data?.action === 'deny') { // deny login only if return 200 & action = deny.
// Custom actions
}
} catch (error) {
console.log(error);
}
};
We need to add a dependency to Axios, as we use it as the HTTP client.
- Click on Add Dependency
- Enter
axios
in the name field and click on Create
- Configuration is now done and you can deploy this new action to your tenant
- Click on Deploy
Now you have to use the new action (Account Protect - Registration) in your registration pipeline.
-
Go to Actions > Flows
-
Click on Login
-
Click on Custom on the right panel and move action Account Protect Registration in your pipeline
-
Click on Apply
From now on, you will send all registrations to DataDome Account Protect.
Step 3: Configure Auth0 to send failed login events to DataDome Account Protect
You need to configure an Auth0 stream to a DataDome webhook.
- Go to Monitoring > Streams and click on Create Stream
- Enter the following information
- Name: Account Protect - Failed Login
- Payload URL: https://account-api.datadome.co/v1/collect/auth0/log-stream
- Authorization Token: The Account Protect Key available on your Dashboard
- Content Type: application/json
- Content Format:JSON Object
- Filter by Event Category: Select Login - Failure
- Don't forget to click on "Apply" to ensure it is taken into account
- Click on Save
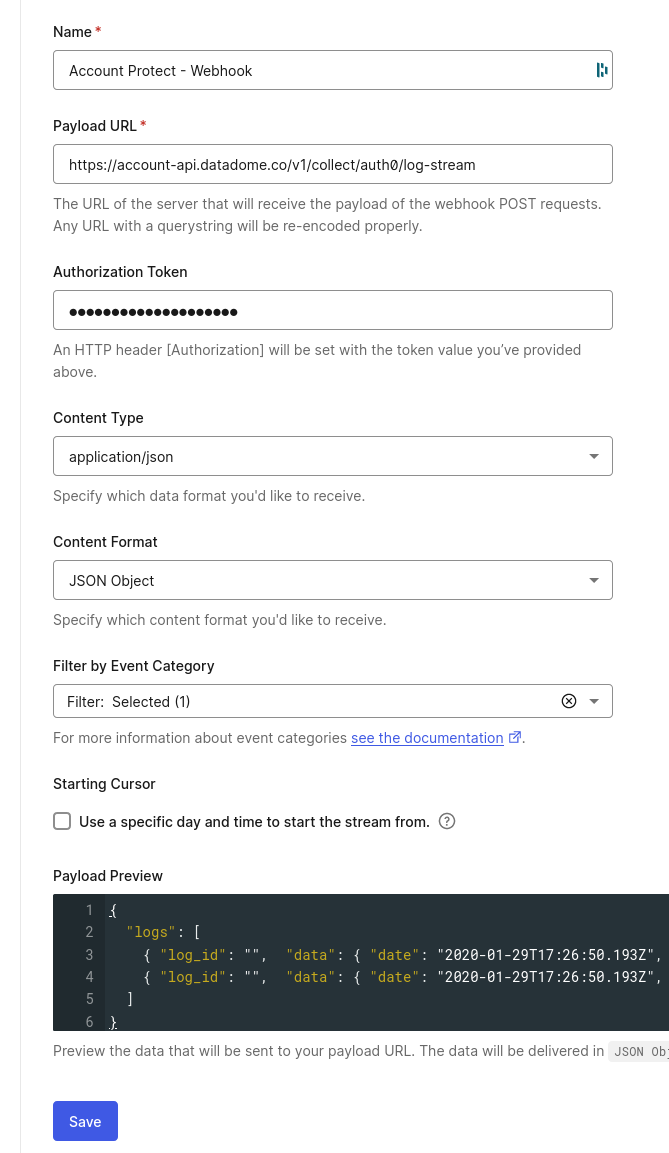
From now on, you will send all failed login events to DataDome Account Protect.
How to check failed login events that were not sent to DataDome Account Protect
- Go to Monitoring > Streams and click on Account Protect - Failed Login
- Select the Health tab
This will allow you to check the last errors received by the Auth0 webhook.
In the example below, the wrong API key was used:
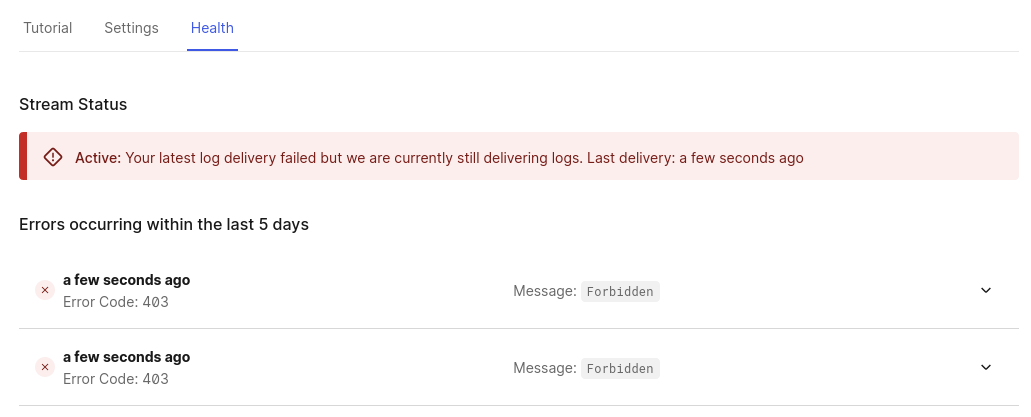
Updated 4 months ago